This article provides the basics of docker and learn how to build an image that runs a Python application in a container. I will show you how to build a custom application for Sales reps – SAP Commissions.
Customers/Developers in your org can build your own custom Application to help Sales reps to see what they need to maximize the sales… you can build this all through the data coming from Rest APIs..
What is Docker?
It is a platform for developing, shipping, and running applications. In simple terms, you can build your application, package them along with their dependencies into a container, and then these containers can be shipped to run on other machines.
Key Terminologies
- Dockerfile– A text file that consists of instructions and arguments. It informs Docker how the image should get built.
- Image- It is a read-only template with instructions for creating a container. Each instruction in a Dockerfile creates a layer in the image.
- Container- It is a running instance of an image. It is well defined by its image as well as any configuration options provided to it create or start.
The above diagram shows multiple running instances (container) of the same image (template) which was created or built using a Dockerfile.
The most commonly used Dockerfile instructions are :
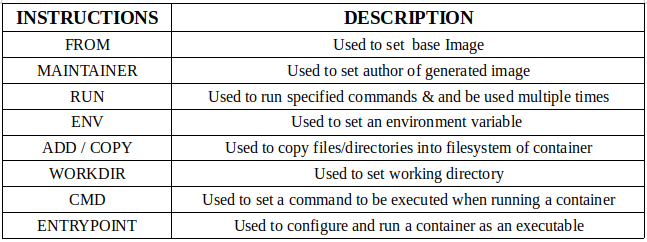
Docker Commands
The most commonly used docker commands are :

Get Started to build sample SAP Commissions Application
We will create a simple project to containerize a Python application by following the steps mentioned below :
- Create a simple static web application using flask
- Create a Dockerfile
- Build image out of Dockerfile
- Run the image in the container
- Push the image to Docker Hub
- Deploy the application to SAP BTP
Installation
- Download the Docker (requires a paid subscription.) or you can choose Rancher Desktop or Podman
- Install the pip library required to build for your application
Code
app.py
from flask import Flask, render_template
import os
app = Flask(__name__)
@app.route('/')
def home():
return render_template('home.html')
if __name__ == '__main__':
app.run(debug=True,host='0.0.0.0')
requirements.txt
flask
requests
json
pandas
Dockerfile
FROM python:3.10
WORKDIR /app
COPY . .
RUN pip install -r requirements.txt
CMD ["python","app.py"]
Run
Open terminal and navigate to the project .
- Build an image out of Dockerfile by running the following command below. Pass in the
-t
parameter to name the image .
docker build -t sample .
To verify the image built use the following command
docker images
Run the docker container . The -p
flag maps a port running inside the container to your host. The — — name is used to give name to the container and sample is image name which was built earlier .
docker run --name flask_app -p 8000:5000 sample
Open the browser and type to see your application running locally to see the preview
http:://127.0.0.1:8000/
Above is the custom application developed in this example for Sales reps to show in One Page about the Sales Performance and how the Sales transactions are in his geography to identify the potential sales area.
Login to your Docker Hub to push your image
docker login -u <username>
docker build -t <username>/image-name .
docker push <username>/image-name:latest
docker run <username>/image-name
Push Docker Image to SAP BTP
Now the image is ready in the docker hub, let’s push this docker image to SAP BTP.
Here I assume that you have your SAP BTP Trial or Global account ready and Cloud Foundry Command Line Interface (CLI) installed on your desktop.
https://account.hanatrial.ondemand.com/
Step1: Let’s login to your SAP BTP Cloud Foundry endpoint using CLI. Run:
cf login --sso
Step2: Push the docker image to SAP Cloud Foundry.
cf push custom-application --docker-image <username>/image-name --docker-username <username>
Example for the command line syntax
cf push <App Name> –docker-image <Docker Image Repository:TagName> –docker- username <docker username>
End Result
Now, you have pretty much an Idea of building your own Application by consuming the data from SAP Commissions APIs .. Try yourself, in case you face any issue or have questions, leave your comment below.
Thanks for reading!
Don’t forget to share this article with your friends or colleagues.
Feel free to connect with me on any of the platforms below! 🚀
Yogananda Muthaiah |Twitter | LinkedIn | GitHub
Docker Architecture for reference
Watch out how to develop a simple Front End application